This is an article to show you how to, using Deluge code, generate all the email signatures in the user profile in ZohoCRM.
Why?
To simplify the on-boarding process. A client of ours has a rather rich HTML email signature with a profile photo included. When sending an email from CRM, they want CRM to include this rich signature (different per user) at the bottom of the email message.
How?
So there are a few issues with displaying images which would have other solutions, such as hosting the images on a web-server, or converting to a base64 or an SVG. ZohoCRM signatures seem to be unable to handle a lot of code or long URLs such as a base64 source URL for an image.
The Plan
- Get company phone and website from Organization Details in ZohoCRM.
- Upload and get publicly-accessible image URL to/from ZohoWriter.
- Loop through active users in ZohoCRM.
- Merge the HTML email signature with the fields evaluated.
Function: fn_Workdrive_HostImage
I add this function to the CRM and use it as a standalone function to upload generic images (such as facebook, instagram, icons, and partner logos). This function is for images that will be on all signatures and don't have anything identifying per user. So this function uploads the image to the client's workdrive and you will need the long hash ID of the folder:
- Login as the client to ZohoWorkdrive (www.zoho.com/workdrive)
- Signin or Access
- You should be in "My Folders" (left sidebar)
- Create a folder, I'm calling mine "Email Signature Images"
- Within that folder, create another folder called "_Global".
- At the same level, create another folder called "Profile Photos".
- Navigate into the "_Global" folder and note the last hash in the URL (after "/folders/")
/* ******************************************************************************* Function: String fn_Workdrive_HostImage(string p_DownloadImageUrl, string p_WorkdriveFolder) Label: Fn - Workdrive - Host Image Trigger: Off a button / Standalone Purpose: This uploads an image to workdrive and returns a publicly-accessible link/URL for use in your email signatures. Inputs: Loginuser Outputs: Email Scope(s): WorkDrive.team.ALL, WorkDrive.workspace.ALL, WorkDrive.files.ALL, WorkDrive.organization.READ, WorkDrive.teamfolders.ALL, WorkDrive.links.ALL, WorkDrive.DataTemplates.READ Date Created: 2023-12-05 (Joel Lipman) - Initial release - Uploads an image to workdrive and returns the public link Date Modified: ??? - ??? ttps://workdrive.zoho.com/apidocs/v1/filefoldersharing/shareeveryone ******************************************************************************* */ // // Go create a folder in workdrive, take the last hash string after the word /folders/ in the URL: v_TargetFolder = ifnull(p_WorkdriveFolder,"abcdefghijklmnopqrstuvwxyz12345678901"); v_ImageToDownload = ifnull(p_DownloadImageUrl,"https://joellipman.com/tmp/icon_linkedin.jpg"); v_FileName = v_ImageToDownload.subString(v_ImageToDownload.lastIndexOf("/") + 1); // // get file object f_File = invokeurl [ url :v_ImageToDownload type :GET ]; // // upload file to workdrive r_WorkdriveUpload = zoho.workdrive.uploadFile(f_File,v_TargetFolder,v_FileName,true,"zworkdrive"); info "Workdrive response:"; info r_WorkdriveUpload; if(!isNull(r_WorkdriveUpload.get("data"))) { // // set permissions to public and visible m_Headers = Map(); m_Headers.put("Accept","application/vnd.api+json"); for each r_Data in r_WorkdriveUpload.get("data") { r_Attributes = r_Data.get("attributes"); v_ResourceID = r_Attributes.get("resource_id"); } // // generate permissions to send to workdrive m_Attributes = Map(); m_Attributes.put("resource_id",v_ResourceID); m_Attributes.put("shared_type","everyone"); // 34 for files // 6 for folders m_Attributes.put("role_id","34"); m_Data = Map(); m_Data.put("attributes",m_Attributes); m_Data.put("type","permissions"); m_Params = Map(); m_Params.put("data",m_Data); r_Permissions = invokeurl [ url :"https://www.zohoapis.eu/workdrive/api/v1/permissions" type :POST parameters:m_Params.toString() headers:m_Headers connection:"zworkdrive" ]; info "Workdrive Permissions:"; info r_Permissions; // // response returns public permalink if(!isNull(r_Permissions.get("data"))) { if(!isNull(r_Permissions.get("data").get("attributes"))) { v_ImageURL = r_Permissions.get("data").get("attributes").get("permalink"); v_ImageURL = v_ImageURL.replaceAll("https://workdrive.zohopublic.eu/file/","",true); // // replaced with URL taken on inspect element v_ImageURL = "https://previewengine-accl.zohopublic.eu/image/WD/" + v_ImageURL; } } } return v_ImageURL;
- /* *******************************************************************************
- Function: string fn_Workdrive_HostImage(string p_DownloadImageUrl, string p_WorkdriveFolder)
- Label: Fn - Workdrive - Host Image
- Trigger: Off a button / Standalone
- Purpose: This uploads an image to workdrive and returns a publicly-accessible link/URL for use in your email signatures.
- Inputs: Loginuser
- Outputs: Email
- Scope(s): WorkDrive.team.ALL, WorkDrive.workspace.ALL, WorkDrive.files.ALL, WorkDrive.organization.READ, WorkDrive.teamfolders.ALL, WorkDrive.links.ALL, WorkDrive.DataTemplates.READ
- Date Created: 2023-12-05 (Joel Lipman)
- - Initial release
- - Uploads an image to workdrive and returns the public link
- Date Modified: ???
- - ???
- ttps://workdrive.zoho.com/apidocs/v1/filefoldersharing/shareeveryone
- ******************************************************************************* */
- //
- // Go create a folder in workdrive, take the last hash string after the word /folders/ in the url:
- v_TargetFolder = ifnull(p_WorkdriveFolder,"abcdefghijklmnopqrstuvwxyz12345678901");
- v_ImageToDownload = ifnull(p_DownloadImageUrl,"https://joellipman.com/tmp/icon_linkedin.jpg");
- v_FileName = v_ImageToDownload.subString(v_ImageToDownload.lastIndexOf("/") + 1);
- //
- // get file object
- f_File = invokeUrl
- [
- url :v_ImageToDownload
- type :GET
- ];
- //
- // upload file to workdrive
- r_WorkdriveUpload = zoho.workdrive.uploadFile(f_File,v_TargetFolder,v_FileName,true,"zworkdrive");
- info "Workdrive response:";
- info r_WorkdriveUpload;
- if(!isNull(r_WorkdriveUpload.get("data")))
- {
- //
- // set permissions to public and visible
- m_Headers = Map();
- m_Headers.put("Accept","application/vnd.api+json");
- for each r_Data in r_WorkdriveUpload.get("data")
- {
- r_Attributes = r_Data.get("attributes");
- v_ResourceID = r_Attributes.get("resource_id");
- }
- //
- // generate permissions to send to workdrive
- m_Attributes = Map();
- m_Attributes.put("resource_id",v_ResourceID);
- m_Attributes.put("shared_type","everyone");
- // 34 for files // 6 for folders
- m_Attributes.put("role_id","34");
- m_Data = Map();
- m_Data.put("attributes",m_Attributes);
- m_Data.put("type","permissions");
- m_Params = Map();
- m_Params.put("data",m_Data);
- r_Permissions = invokeUrl
- [
- url :"https://www.zohoapis.eu/workdrive/api/v1/permissions"
- type :POST
- parameters:m_Params.toString()
- headers:m_Headers
- connection:"zworkdrive"
- ];
- info "Workdrive Permissions:";
- info r_Permissions;
- //
- // response returns public permalink
- if(!isNull(r_Permissions.get("data")))
- {
- if(!isNull(r_Permissions.get("data").get("attributes")))
- {
- v_ImageURL = r_Permissions.get("data").get("attributes").get("permalink");
- v_ImageURL = v_ImageURL.replaceAll("https://workdrive.zohopublic.eu/file/","",true);
- //
- // replaced with URL taken on inspect element
- v_ImageURL = "https://previewengine-accl.zohopublic.eu/image/WD/" + v_ImageURL;
- }
- }
- }
- return v_ImageURL;
Function: fn_Settings_EmailSignatures
Important: the following code will overwrite ALL signatures in the CRM. The email signature it will overwrite is the one held in ZohoCRM > Setup > Channels > Email > Email Configuration > Compose > Email Signature as in the following screenshot:
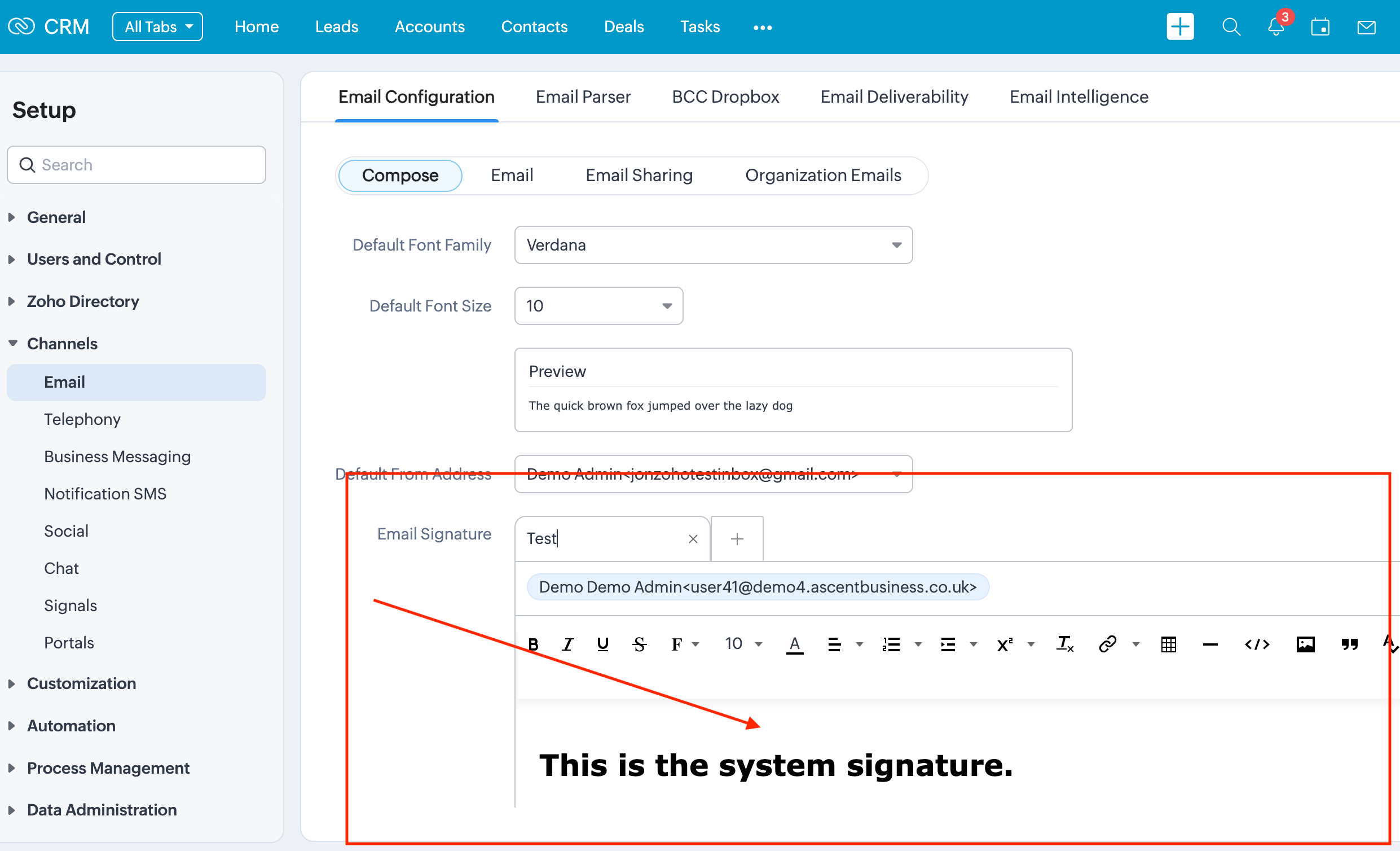
/* ******************************************************************************* Function: String fn_Settings_GenerateEmailSignatures() Label: Fn - Settings - Generate Email Signatures Trigger: Off a button / Standalone / Schedule to run daily @ 1am Purpose: Generates the email signature for all active users on their profile into the CRM settings. Inputs: - Outputs: - Scope(s): WorkDrive.team.ALL, WorkDrive.workspace.ALL, WorkDrive.files.ALL, WorkDrive.organization.READ, WorkDrive.teamfolders.ALL, WorkDrive.links.ALL, WorkDrive.DataTemplates.READ ZohoCRM.modules.ALL, ZohoCRM.org.READ, ZohoCRM.settings.ALL, ZohoCRM.users.ALL, ZohoCRM.notifications.ALL, ZohoCRM.Files.CREATE, ZohoCRM.coql.READ, ZohoCRM.Files.READ Date Created: 2023-12-04 (Joel Lipman) - Initial release - Generates an email signature using the photo on the profile. Date Modified: ??? - ??? https://workdrive.zoho.com/apidocs/v1/filefoldersharing/shareeveryone ******************************************************************************* */ l_PhoneString = List(); v_CompanyWebsite = ""; // // get org details m_Blank = Map(); m_OrgDetails = zoho.crm.invokeConnector("crm.getorg",m_Blank); info m_OrgDetails.get("response"); if(!isNull(m_OrgDetails.get("response"))) { if(!isNull(m_OrgDetails.get("response").get("org"))) { l_OrgDetails = m_OrgDetails.get("response").get("org"); for each m_OrgDetail in l_OrgDetails { if(!isNull(m_OrgDetail.get("phone"))) { l_PhoneString.add(m_OrgDetail.get("phone")); } if(!isNull(m_OrgDetail.get("website"))) { v_CompanyWebsite = m_OrgDetail.get("website"); } } } } // // run fn_Workdrive_HostImage function to get these src URLs: v_Icon_TwitterHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890a\" style=\"width:15px;height:15px;margin:0;\" alt=\"Facebook\" />"; v_Icon_LinkedInHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890b\" style=\"width:15px;height:15px;margin:0;\" alt=\"LinkedIn\" />"; v_Icon_PinterestHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890c\" style=\"width:15px;height:15px;margin:0;\" alt=\"Instagram\" />"; v_Logos_PartnersHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890d\" style=\"width:300px;height:auto;margin:0;\" alt=\"Partners\" />"; // // the generic signature for all users with merge fields enclosed with square tags: v_RecodedSignature = "<table style=\"max-width:640px;font-family:Arial, Helvetica, sans-serif\"> <tr> <td rowspan=\"2\" style=\"max-width:160px;\"><img src=\"[AB-Image]\" style=\"width:150px;height:150px;\" alt=\"[AB-StaffName]\" /></td> <td style=\"min-width:250px;line-height:12pt;\"> <span style=\"font-weight:600;color:#005339;font-size: 12pt;\">[AB-StaffName]</span><br /> <span style=\"font-weight:600;color:#efdb00;font-size: 12pt;\">[AB-JobTitle]</span><br /> <span style=\"font-weight:700;color:#005339;font-size: 10pt;\">[AB-MobilePhone]</span><br /><a href=\"mailto:[AB-Email]\" style=\"text-decoration: none;\"><span style=\"font-weight:700;color:#005339;font-size: 10pt;text-transform:lowercase\">[AB-Email]</span></a><br /><a href=\"https://www.joellipman.com\" style=\"text-decoration: none;\"><span style=\"font-weight:700;color:#005339;font-size: 10pt;\">www.joellipman.com</span></a><br /> <div style=\"border-top:3px solid #efdb00;margin: 5px 0;padding:5px 0;\"> <a href=\"https://twitter.com/joel_lipman\">" + v_Icon_TwitterHTML + "</a> <a href=\"https://uk.linkedin.com/in/joelipman/\">" + v_Icon_LinkedInHTML + "</a> <a href=\"https://www.pinterest.co.uk/Joel_Lipman\">" + v_Icon_PinterestHTML + "</a> </div> </td> <td style=\"vertical-align: bottom;\"> " + v_Logos_PartnersHTML + " </td> </tr><tr> <td colspan=\"3\" style=\"text-align:justify;font-size:7pt;color:#ccc;line-height:10pt;padding:5px;\"> <p> <b>Important Terms & Conditions:</b><br /> <br /> <i>By choosing to purchase or hire ... </p> </td> </tr> </table>"; // // get all active users in CRM m_UserMapping = Map(); v_Endpoint = "https://www.zohoapis.eu/crm/v4/users?type=ActiveUsers"; r_Users = invokeurl [ url :v_Endpoint type :GET connection:"zcrm" ]; l_UserUpdates = List(); for each m_User in r_Users.get("users") { v_CurrentSignature = ifnull(m_User.get("signature"),""); // // criteria, only update signatures which aren't done? //if(v_CurrentSignature.length() < 1) //{ // v_ImageURL = ""; if(!isNull(m_User.get("Signature_Image"))) { for each m_SignatureImage in m_User.get("Signature_Image") { if(!isNull(m_SignatureImage.get("file_Id"))) { // // get file object f_File = invokeurl [ url :"https://www.zohoapis.eu/crm/v4/files?id=" + m_SignatureImage.get("file_Id") type :GET connection:"zcrm" ]; // // let's rename the file the name of the staff member along with the file type v_FileName = m_User.get("full_name"); v_FileExtension = m_SignatureImage.get("extn"); v_FileNameFull = v_FileName + "." + v_FileExtension; // // upload to profile photos folder (enter the workdrive last hash noted earlier) v_StaticFolder = "abcdefghijklmnopqrstuvwxyz12345678901"; // // upload file to workdrive r_WorkdriveUpload = zoho.workdrive.uploadFile(f_File,v_StaticFolder,v_FileNameFull,true,"zworkdrive"); info "Workdrive response:"; info r_WorkdriveUpload; if(!isNull(r_WorkdriveUpload.get("data"))) { // // set permissions to public and visible m_Headers = Map(); m_Headers.put("Accept","application/vnd.api+json"); for each r_Data in r_WorkdriveUpload.get("data") { r_Attributes = r_Data.get("attributes"); v_ResourceID = r_Attributes.get("resource_id"); } // // generate permissions to send to workdrive m_Attributes = Map(); m_Attributes.put("resource_id",v_ResourceID); m_Attributes.put("shared_type","everyone"); // 34 files // 6 for folders m_Attributes.put("role_id","34"); m_Data = Map(); m_Data.put("attributes",m_Attributes); m_Data.put("type","permissions"); m_Params = Map(); m_Params.put("data",m_Data); r_Permissions = invokeurl [ url :"https://www.zohoapis.eu/workdrive/api/v1/permissions" type :POST parameters:m_Params.toString() headers:m_Headers connection:"zworkdrive" ]; info "Workdrive Permissions:"; info r_Permissions; // // response returns public permalink if(!isNull(r_Permissions.get("data"))) { if(!isNull(r_Permissions.get("data").get("attributes"))) { v_ImageURL = r_Permissions.get("data").get("attributes").get("permalink"); v_ImageURL = v_ImageURL.replaceAll("https://workdrive.zohopublic.eu/file/","",true); // // replaced with URL taken on inspect element v_ImageURL = "https://previewengine-accl.zohopublic.eu/image/WD/" + v_ImageURL; } } } } } } // v_Name = ifNull(m_User.get("full_name"),""); v_JobTitle = ifNull(m_User.get("Signature_Job_Title"),""); v_Phone = ifNull(ifNull(m_User.get("phone"),m_User.get("mobile")),""); v_Email = ifNull(m_User.get("email"),""); // l_PhoneString.add(v_Phone); v_PhoneStr = l_PhoneString.toString(" / "); // // merge with generic signature v_ThisSig = v_RecodedSignature; v_ThisSig = v_ThisSig.replaceAll("[AB-Image]",v_ImageURL,true); v_ThisSig = v_ThisSig.replaceAll("[AB-StaffName]",v_Name,true); v_ThisSig = v_ThisSig.replaceAll("[AB-JobTitle]",v_JobTitle,true); v_ThisSig = v_ThisSig.replaceAll("[AB-MobilePhone]",v_PhoneStr,true); v_ThisSig = v_ThisSig.replaceAll("[AB-Email]",v_Email,true); // // update signature on user profile m_UserSignature = Map(); m_UserSignature.put("id",m_User.get("id")); m_UserSignature.put("signature",v_ThisSig); l_UserUpdates.add(m_UserSignature); //} } // // if updates exist then update the users module with these new signatures if(l_UserUpdates.size() > 0) { m_Params = Map(); m_Params.put("users",l_UserUpdates); r_UserUpdates = invokeurl [ url :"https://www.zohoapis.eu/crm/v4/users" type :PUT parameters:m_Params.toString() connection:"zcrm" ]; info r_UserUpdates; } return "";
- /* *******************************************************************************
- Function: string fn_Settings_GenerateEmailSignatures()
- Label: Fn - Settings - Generate Email Signatures
- Trigger: Off a button / Standalone / Schedule to run daily @ 1am
- Purpose: Generates the email signature for all active users on their profile into the CRM settings.
- Inputs: -
- Outputs: -
- Scope(s): WorkDrive.team.ALL, WorkDrive.workspace.ALL, WorkDrive.files.ALL, WorkDrive.organization.READ, WorkDrive.teamfolders.ALL, WorkDrive.links.ALL, WorkDrive.DataTemplates.READ
- ZohoCRM.modules.ALL, ZohoCRM.org.READ, ZohoCRM.settings.ALL, ZohoCRM.users.ALL, ZohoCRM.notifications.ALL, ZohoCRM.Files.CREATE, ZohoCRM.coql.READ, ZohoCRM.Files.READ
- Date Created: 2023-12-04 (Joel Lipman)
- - Initial release
- - Generates an email signature using the photo on the profile.
- Date Modified: ???
- - ???
- https://workdrive.zoho.com/apidocs/v1/filefoldersharing/shareeveryone
- ******************************************************************************* */
- l_PhoneString = List();
- v_CompanyWebsite = "";
- //
- // get org details
- m_Blank = Map();
- m_OrgDetails = zoho.crm.invokeConnector("crm.getorg",m_Blank);
- info m_OrgDetails.get("response");
- if(!isNull(m_OrgDetails.get("response")))
- {
- if(!isNull(m_OrgDetails.get("response").get("org")))
- {
- l_OrgDetails = m_OrgDetails.get("response").get("org");
- for each m_OrgDetail in l_OrgDetails
- {
- if(!isNull(m_OrgDetail.get("phone")))
- {
- l_PhoneString.add(m_OrgDetail.get("phone"));
- }
- if(!isNull(m_OrgDetail.get("website")))
- {
- v_CompanyWebsite = m_OrgDetail.get("website");
- }
- }
- }
- }
- //
- // run fn_Workdrive_HostImage function to get these src URLs:
- v_Icon_TwitterHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890a\" style=\"width:15px;height:15px;margin:0;\" alt=\"Facebook\" />";
- v_Icon_LinkedInHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890b\" style=\"width:15px;height:15px;margin:0;\" alt=\"LinkedIn\" />";
- v_Icon_PinterestHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890c\" style=\"width:15px;height:15px;margin:0;\" alt=\"Instagram\" />";
- v_Logos_PartnersHTML = "<img src=\"https://previewengine-accl.zohopublic.eu/image/WD/abcdefghijklmnopqrstuvwxyz1234567890d\" style=\"width:300px;height:auto;margin:0;\" alt=\"Partners\" />";
- //
- // the generic signature for all users with merge fields enclosed with square tags:
- v_RecodedSignature = "<table style=\"max-width:640px;font-family:Arial, Helvetica, sans-serif\"> <tr> <td rowspan=\"2\" style=\"max-width:160px;\"><img src=\"[AB-Image]\" style=\"width:150px;height:150px;\" alt=\"[AB-StaffName]\" /></td> <td style=\"min-width:250px;line-height:12pt;\"> <span style=\"font-weight:600;color:#005339;font-size: 12pt;\">[AB-StaffName]</span><br /> <span style=\"font-weight:600;color:#efdb00;font-size: 12pt;\">[AB-JobTitle]</span><br /> <span style=\"font-weight:700;color:#005339;font-size: 10pt;\">[AB-MobilePhone]</span><br /><a href=\"mailto:[AB-Email]\" style=\"text-decoration: none;\"><span style=\"font-weight:700;color:#005339;font-size: 10pt;text-transform:lowercase\">[AB-Email]</span></a><br /><a href=\"https://www.joellipman.com\" style=\"text-decoration: none;\"><span style=\"font-weight:700;color:#005339;font-size: 10pt;\">www.joellipman.com</span></a><br /> <div style=\"border-top:3px solid #efdb00;margin: 5px 0;padding:5px 0;\"> <a href=\"https://twitter.com/joel_lipman\">" + v_Icon_TwitterHTML + "</a> <a href=\"https://uk.linkedin.com/in/joelipman/\">" + v_Icon_LinkedInHTML + "</a> <a href=\"https://www.pinterest.co.uk/Joel_Lipman\">" + v_Icon_PinterestHTML + "</a> </div> </td> <td style=\"vertical-align: bottom;\"> " + v_Logos_PartnersHTML + " </td> </tr><tr> <td colspan=\"3\" style=\"text-align:justify;font-size:7pt;color:#ccc;line-height:10pt;padding:5px;\"> <p> <b>Important Terms & Conditions:</b><br /> <br /> <i>By choosing to purchase or hire ... </p> </td> </tr> </table>";
- //
- // get all active users in CRM
- m_UserMapping = Map();
- v_Endpoint = "https://www.zohoapis.eu/crm/v4/users?type=ActiveUsers";
- r_Users = invokeUrl
- [
- url :v_Endpoint
- type :GET
- connection:"zcrm"
- ];
- l_UserUpdates = List();
- for each m_User in r_Users.get("users")
- {
- v_CurrentSignature = ifnull(m_User.get("signature"),"");
- //
- // criteria, only update signatures which aren't done?
- //if(v_CurrentSignature.length() < 1)
- //{
- //
- v_ImageURL = "";
- if(!isNull(m_User.get("Signature_Image")))
- {
- for each m_SignatureImage in m_User.get("Signature_Image")
- {
- if(!isNull(m_SignatureImage.get("file_Id")))
- {
- //
- // get file object
- f_File = invokeUrl
- [
- url :"https://www.zohoapis.eu/crm/v4/files?id=" + m_SignatureImage.get("file_Id")
- type :GET
- connection:"zcrm"
- ];
- //
- // let's rename the file the name of the staff member along with the file type
- v_FileName = m_User.get("full_name");
- v_FileExtension = m_SignatureImage.get("extn");
- v_FileNameFull = v_FileName + "." + v_FileExtension;
- //
- // upload to profile photos folder (enter the workdrive last hash noted earlier)
- v_StaticFolder = "abcdefghijklmnopqrstuvwxyz12345678901";
- //
- // upload file to workdrive
- r_WorkdriveUpload = zoho.workdrive.uploadFile(f_File,v_StaticFolder,v_FileNameFull,true,"zworkdrive");
- info "Workdrive response:";
- info r_WorkdriveUpload;
- if(!isNull(r_WorkdriveUpload.get("data")))
- {
- //
- // set permissions to public and visible
- m_Headers = Map();
- m_Headers.put("Accept","application/vnd.api+json");
- for each r_Data in r_WorkdriveUpload.get("data")
- {
- r_Attributes = r_Data.get("attributes");
- v_ResourceID = r_Attributes.get("resource_id");
- }
- //
- // generate permissions to send to workdrive
- m_Attributes = Map();
- m_Attributes.put("resource_id",v_ResourceID);
- m_Attributes.put("shared_type","everyone");
- // 34 files // 6 for folders
- m_Attributes.put("role_id","34");
- m_Data = Map();
- m_Data.put("attributes",m_Attributes);
- m_Data.put("type","permissions");
- m_Params = Map();
- m_Params.put("data",m_Data);
- r_Permissions = invokeUrl
- [
- url :"https://www.zohoapis.eu/workdrive/api/v1/permissions"
- type :POST
- parameters:m_Params.toString()
- headers:m_Headers
- connection:"zworkdrive"
- ];
- info "Workdrive Permissions:";
- info r_Permissions;
- //
- // response returns public permalink
- if(!isNull(r_Permissions.get("data")))
- {
- if(!isNull(r_Permissions.get("data").get("attributes")))
- {
- v_ImageURL = r_Permissions.get("data").get("attributes").get("permalink");
- v_ImageURL = v_ImageURL.replaceAll("https://workdrive.zohopublic.eu/file/","",true);
- //
- // replaced with URL taken on inspect element
- v_ImageURL = "https://previewengine-accl.zohopublic.eu/image/WD/" + v_ImageURL;
- }
- }
- }
- }
- }
- }
- //
- v_Name = ifNull(m_User.get("full_name"),"");
- v_JobTitle = ifNull(m_User.get("Signature_Job_Title"),"");
- v_Phone = ifNull(ifNull(m_User.get("phone"),m_User.get("mobile")),"");
- v_Email = ifNull(m_User.get("email"),"");
- //
- l_PhoneString.add(v_Phone);
- v_PhoneStr = l_PhoneString.toString(" / ");
- //
- // merge with generic signature
- v_ThisSig = v_RecodedSignature;
- v_ThisSig = v_ThisSig.replaceAll("[AB-Image]",v_ImageURL,true);
- v_ThisSig = v_ThisSig.replaceAll("[AB-StaffName]",v_Name,true);
- v_ThisSig = v_ThisSig.replaceAll("[AB-JobTitle]",v_JobTitle,true);
- v_ThisSig = v_ThisSig.replaceAll("[AB-MobilePhone]",v_PhoneStr,true);
- v_ThisSig = v_ThisSig.replaceAll("[AB-Email]",v_Email,true);
- //
- // update signature on user profile
- m_UserSignature = Map();
- m_UserSignature.put("id",m_User.get("id"));
- m_UserSignature.put("signature",v_ThisSig);
- l_UserUpdates.add(m_UserSignature);
- //}
- }
- //
- // if updates exist then update the users module with these new signatures
- if(l_UserUpdates.size() > 0)
- {
- m_Params = Map();
- m_Params.put("users",l_UserUpdates);
- r_UserUpdates = invokeUrl
- [
- url :"https://www.zohoapis.eu/crm/v4/users"
- type :PUT
- parameters:m_Params.toString()
- connection:"zcrm"
- ];
- info r_UserUpdates;
- }
- return "";
Source(s):